Часть 1



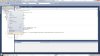
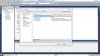

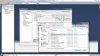

#ADE32.h
Код:
//==================================================================
// This file is part of Qmo Base D3D Class part 2
// (c) copyright Qmo 2011™
// special thanks to : mpgh.net
//==================================================================
#ifndef __FAKE_H__
#define __FAKE_H__
#define C_ERROR 0xFFFFFFFF
#define C_ADDR1 0x00000001
#define C_ADDR2 0x00000002
#define C_ADDR4 0x00000004
#define C_LOCK 0x00000008
#define C_67 0x00000010
#define C_66 0x00000020
#define C_REP 0x00000040
#define C_SEG 0x00000080
#define C_ANYPREFIX (C_66+C_67+C_LOCK+C_REP+C_SEG)
#define C_DATA1 0x00000100
#define C_DATA2 0x00000200
#define C_DATA4 0x00000400
#define C_SIB 0x00000800
#define C_ADDR67 0x00001000
#define C_DATA66 0x00002000
#define C_MODRM 0x00004000
#define C_BAD 0x00008000
#define C_OPCODE2 0x00010000
#define C_REL 0x00020000
#define C_STOP 0x00040000
#pragma pack(push)
#pragma pack(1)
struct disasm_struct {
BYTE disasm_defaddr; // 00
BYTE disasm_defdata; // 01
DWORD disasm_len; // 02 03 04 05
DWORD disasm_flag; // 06 07 08 09
DWORD disasm_addrsize; // 0A 0B 0C 0D
DWORD disasm_datasize; // 0E 0F 10 11
BYTE disasm_rep; // 12
BYTE disasm_seg; // 13
BYTE disasm_opcode; // 14
BYTE disasm_opcode2; // 15
BYTE disasm_modrm; // 16
BYTE disasm_sib; // 17
union {
BYTE disasm_addr_b[8]; // 18 19 1A 1B 1C 1D 1E 1F
WORD disasm_addr_w[4];
DWORD disasm_addr_d[2];
char disasm_addr_c[8];
short disasm_addr_s[4];
long disasm_addr_l[2];
};
union {
BYTE disasm_data_b[8]; // 20 21 22 23 24 25 26 27
WORD disasm_data_w[4];
DWORD disasm_data_d[2];
char disasm_data_c[8];
short disasm_data_s[4];
long disasm_data_l[2];
};
};
#pragma pack(pop)
int disasm(BYTE* opcode0, disasm_struct* diza);
int oplen(BYTE* opcode);
#endif
/**************************************************************************************************************************************************************************************************************/
Код:
//-----------------------------------------------------------------------------
// Copyright © November/2011. MPGH/Qmoa. All rights reserved.
// || Do not forget to credits ||
//-----------------------------------------------------------------------------
// flavor from the Base cheat ... need to Edit ok, and the creation of your abilities
//( Clue : discard the unnecessary )
//-----------------------------------------------------------------------------
// To Be only Included in cBase.cpp Otherwise needs Modification to Provide Cross object linking
#include "stdafx.h"
#pragma once
class cBase
{
public:
FILE *LOG;
char dllpath[255];
char logfile[255];
struct sscreen
{
int height;
int width;
}screen;
char* GetFile(char *file);
void WriteFile(FILE *file,const char *fmt, ...);
};
extern cBase Base;
Код:
//-----------------------------------------------------------------------------
// Copyright © November/2011. MPGH/Qmoa. All rights reserved.
// || Do not forget to credits ||
//-----------------------------------------------------------------------------
// flavor from the Base cheat ... need to Edit ok, and the creation of your abilities
//( Clue : discard the unnecessary )
//-----------------------------------------------------------------------------
#define WIN32_LEAN_AND_MEAN
#define WIN32_EXTRA_LEAN
#include
#include "ADE32.h"
#define DETOUR_LEN_AUTO 0 // Finds the detour length automatically
enum
{
DETOUR_TYPE_JMP, // min detour len: 5
DETOUR_TYPE_PUSH_RET, // min detour len: 6
DETOUR_TYPE_NOP_JMP, // min detour len: 6
DETOUR_TYPE_NOP_NOP_JMP, // min detour len: 7
DETOUR_TYPE_STC_JC, // min detour len: 7
DETOUR_TYPE_CLC_JNC, // min detour len: 7
};
LPVOID DetourCreate(LPVOID lpFuncOrig, LPVOID lpFuncDetour, int patchType, int detourLen=DETOUR_LEN_AUTO);
LPVOID DetourCreate(LPCSTR lpModuleName, LPCSTR lpProcName, LPVOID lpFuncDetour, int patchType, int detourLen=DETOUR_LEN_AUTO);
BOOL DetourRemove(LPVOID lpDetourCreatePtr);
#pragma warning(disable: 4311)
#pragma warning(disable: 4312)
#pragma warning(disable: 4244)
#define DETOUR_MAX_SRCH_OPLEN 64
#define JMP32_SZ 5
#define BIT32_SZ 4
// jmp32 sig
#define SIG_SZ 3
#define SIG_OP_0 0xCC
#define SIG_OP_1 0x90
#define SIG_OP_2 0xC3
static DWORD Silamguard;
int GetDetourLen(int patchType);
int GetDetourLenAuto(PBYTE &pbFuncOrig, int minDetLen);
// Thin wrapper for APIs
LPVOID DetourCreate(LPCSTR lpModuleName, LPCSTR lpProcName, LPVOID lpFuncDetour, int patchType, int detourLen)
{
LPVOID lpFuncOrig = NULL;
if((lpFuncOrig = GetProcAddress(GetModuleHandle(lpModuleName), lpProcName)) == NULL)
return NULL;
return DetourCreate(lpFuncOrig, lpFuncDetour, patchType, detourLen);
}
LPVOID DetourCreate(LPVOID lpFuncOrig, LPVOID lpFuncDetour, int patchType, int detourLen)
{
LPVOID lpMallocPtr = NULL;
DWORD dwProt = NULL;
PBYTE NewocPBrs = NULL;
PBYTE pbFuncOrig = (PBYTE)lpFuncOrig;
PBYTE pbFuncDetour = (PBYTE)lpFuncDetour;
PBYTE pbPatchBuf = NULL;
int minDetLen = 0;
int detLen = 0;
// Get detour length
if((minDetLen = GetDetourLen(patchType)) == 0)
return NULL;
if(detourLen != DETOUR_LEN_AUTO)
detLen = detourLen;
else if((detLen = GetDetourLenAuto(pbFuncOrig, minDetLen)) < minDetLen)
return NULL;
// Alloc mem for the overwritten bytes
if((lpMallocPtr = (LPVOID)malloc(detLen+JMP32_SZ+SIG_SZ)) == NULL)
return NULL;
NewocPBrs = (PBYTE)lpMallocPtr;
// Enable writing to original
VirtualProtect(lpFuncOrig, detLen, PAGE_READWRITE, &dwProt);
// Write overwritten bytes to the malloc
memcpy(lpMallocPtr, lpFuncOrig, detLen);
NewocPBrs += detLen;
NewocPBrs[0] = 0xE9;
*(DWORD*)(NewocPBrs+1) = (DWORD)((pbFuncOrig+detLen)-NewocPBrs)-JMP32_SZ;
NewocPBrs += JMP32_SZ;
NewocPBrs[0] = SIG_OP_0;
NewocPBrs[1] = SIG_OP_1;
NewocPBrs[2] = SIG_OP_2;
// Create a buffer to prepare the detour bytes
pbPatchBuf = new BYTE[detLen];
memset(pbPatchBuf, 0x90, detLen);
switch(patchType)
{
case DETOUR_TYPE_JMP:
pbPatchBuf[0] = 0xE9;
*(DWORD*)&pbPatchBuf[1] = (DWORD)(pbFuncDetour - pbFuncOrig) - 5;
break;
case DETOUR_TYPE_PUSH_RET:
pbPatchBuf[0] = 0x68;
*(DWORD*)&pbPatchBuf[1] = (DWORD)pbFuncDetour;
pbPatchBuf[5] = 0xC3;
break;
case DETOUR_TYPE_NOP_JMP:
pbPatchBuf[0] = 0x90;
pbPatchBuf[1] = 0xE9;
*(DWORD*)&pbPatchBuf[2] = (DWORD)(pbFuncDetour - pbFuncOrig) - 6;
break;
case DETOUR_TYPE_NOP_NOP_JMP:
pbPatchBuf[0] = 0x90;
pbPatchBuf[1] = 0x90;
pbPatchBuf[2] = 0xE9;
*(DWORD*)&pbPatchBuf[3] = (DWORD)(pbFuncDetour - pbFuncOrig) - 7;
break;
case DETOUR_TYPE_STC_JC:
pbPatchBuf[0] = 0xF9;
pbPatchBuf[1] = 0x0F;
pbPatchBuf[2] = 0x82;
*(DWORD*)&pbPatchBuf[3] = (DWORD)(pbFuncDetour - pbFuncOrig) - 7;
break;
case DETOUR_TYPE_CLC_JNC:
pbPatchBuf[0] = 0xF8;
pbPatchBuf[1] = 0x0F;
pbPatchBuf[2] = 0x83;
*(DWORD*)&pbPatchBuf[3] = (DWORD)(pbFuncDetour - pbFuncOrig) - 7;
break;
default:
return NULL;
}
// Write the detour
for(int i=0; i